Selecting the right interconnect protocol is crucial when designing System-on-Chip (SoC) architectures. AXI (Advanced eXtensible Interface) and AHB (Advanced High-performance Bus) are two widely used protocols from ARM’s AMBA family. Each offers distinct advantages, making them suitable for different applications. We’ll explore their features, performance differences, and use cases while providing updated code examples and user-friendly tables. By the end, you’ll understand which protocol best fits your project.
Understanding AXI vs AHB
Before diving into the AXI vs AHB comparison, let’s clarify what these protocols do. Both AXI and AHB enable communication between components like CPUs, memory, and peripherals in an SoC. However, their designs cater to different needs.
What Is AHB?
AHB, or Advanced High-performance Bus, is an older protocol designed for simpler SoC systems. It prioritizes high-performance data transfers with a single-channel structure. Engineers value AHB for its reliability in less complex designs and legacy systems.
What Is AXI?
AXI, or Advanced eXtensible Interface, is a modern protocol built for high-speed, complex SoCs. It supports multiple channels, burst transfers, and advanced features like outstanding transactions. AXI suits applications demanding high bandwidth and flexibility.
Key Differences Between AXI and AHB
To choose between AXI and AHB, you need to understand their core differences. The table below summarizes their key features, making it easier to compare.
Feature | AXI | AHB |
---|---|---|
Channels | Uses five channels: read address, write address, read data, write data, write response | Uses three channels: address, read data, write data |
Outstanding Transactions | Supports multiple outstanding transactions for reads and writes | Lacks native support; limited to split transactions |
Pipeline Registers | Allows pipeline registers for timing optimization | Does not support pipeline registers |
Throughput | Offers high throughput due to independent channels | Provides limited throughput |
Burst Length | Supports 1–256 (AXI4) or 1–16 (AXI3); burst length known at start | Supports fixed bursts (1, 2, 4, 8, 16) or INCR type |
Quality of Service (QoS) | AXI4 includes QoS support | Lacks QoS support |
Sideband Signals | Supports AxUSER bits for sideband signals | No sideband signal support |
Write Strobes | Supports write strobes for selective data writes | Does not support write strobes |
Power Consumption | Higher due to multiple channels | Lower due to simpler design |
Wiring Complexity | More wires, potentially causing layout congestion | Fewer wires, simpler layout |
This table highlights why AXI often outperforms AHB in modern designs, while AHB remains relevant for simpler systems.
Why AXI Outperforms AHB
AXI generally delivers higher performance than AHB, but why? Let’s explore the two main reasons: duplex transfers and outstanding transactions.
Simplex vs. Duplex Transfers
AXI’s independent read and write channels enable full-duplex communication. This means reads and writes can occur simultaneously, doubling throughput in ideal conditions. For example, a dual-port SRAM slave can process one read and one write in a single clock cycle, maximizing efficiency.
In contrast, AHB uses a simplex model, handling either a read or a write at a time. This limits its performance, especially in systems with simultaneous read/write demands. However, if a system has only one master and one slave, and the slave can’t process reads and writes concurrently, AXI’s advantage diminishes.
Handling Clock Cycle Delays
AXI shines when there are clock delays between a master and a slave, such as in asynchronous clock domains. AXI’s ability to issue outstanding transactions—addresses sent without waiting for data—reduces latency. Once the initial delay passes, data flows continuously, boosting efficiency.
AHB, however, must wait for a response before issuing another transaction. This sequential process slows down performance, especially in systems with clock domain crossing bridges. If no delays exist and only one transaction type (read or write) is used, AXI and AHB perform similarly.
Use Cases for AXI and AHB
Choosing between AXI and AHB depends on your project’s requirements. Here’s a breakdown of their ideal use cases.
When to Use AXI
- High-Performance Systems: AXI suits complex SoCs in smartphones, GPUs, and AI chips requiring high bandwidth.
- Multiple Masters/Slaves: Its support for outstanding transactions and transaction IDs makes AXI ideal for multi-master systems.
- Timing-Critical Designs: Pipeline registers help AXI achieve higher operating frequencies.
- Modern Applications: AXI4’s QoS and sideband signals support advanced features like real-time processing.
When to Use AHB
- Legacy Systems: AHB works well in older designs or systems requiring backward compatibility.
- Simple SoCs: For microcontrollers or low-power devices, AHB’s simplicity reduces design complexity.
- Cost-Sensitive Projects: AHB’s fewer wires and lower power consumption suit budget-conscious designs.
Code Example: AXI vs AHB Implementation
To illustrate the AXI vs AHB difference, let’s examine a simplified Verilog code example for both protocols. The original code has been rewritten with new variable names and a cleaner coding style for clarity.
AXI Master Example
This code demonstrates an AXI master issuing a write transaction with outstanding support.
module axi_master (
input wire clk,
input wire reset_n,
output reg [31:0] wr_addr,
output reg [31:0] wr_data,
output reg wr_valid,
input wire wr_ready,
output reg [3:0] trans_id
);
reg [3:0] state;
localparam IDLE = 4'd0, ADDR = 4'd1, DATA = 4'd2, WAIT = 4'd3;
always @(posedge clk or negedge reset_n) begin
if (!reset_n) begin
state <= IDLE;
wr_addr <= 32'b0;
wr_data <= 32'b0;
wr_valid <= 1'b0;
trans_id <= 4'b0;
end else begin
case (state)
IDLE: begin
if (start_trans) begin
wr_addr <= 32'h1000_0000;
trans_id <= trans_id + 1;
state <= ADDR;
end
end
ADDR: begin
wr_valid <= 1'b1;
if (wr_ready) begin
state <= DATA;
end
end
DATA: begin
wr_data <= 32'hDEAD_BEEF;
if (wr_ready) begin
wr_valid <= 1'b0;
state <= WAIT;
end
end
WAIT: begin
if (resp_received) begin
state <= IDLE;
end
end
endcase
end
end
endmodule
AHB Master Example
This code shows an AHB master performing a single write transaction.
module ahb_master (
input wire hclk,
input wire hreset_n,
output reg [31:0] haddr,
output reg [31:0] hwdata,
output reg hwrite,
output reg [2:0] hburst,
input wire hready
);
reg [2:0] state;
localparam IDLE = 3'd0, ADDR = 3'd1, DATA = 3'd2;
always @(posedge hclk or negedge hreset_n) begin
if (!hreset_n) begin
state <= IDLE;
haddr <= 32'b0;
hwdata <= 32'b0;
hwrite <= 1'b0;
hburst <= 3'b000;
end else begin
case (state)
IDLE: begin
if (init_trans) begin
haddr <= 32'h2000_0000;
hwrite <= 1'b1;
hburst <= 3'b001; // Single burst
state <= ADDR;
end
end
ADDR: begin
if (hready) begin
state <= DATA;
end
end
DATA: begin
hwdata <= 32'hCAFE_1234;
if (hready) begin
hwrite <= 1'b0;
state <= IDLE;
end
end
endcase
end
end
endmodule
These examples highlight AXI’s support for transaction IDs and multiple states, enabling outstanding transactions. AHB’s simpler state machine reflects its sequential nature.
Performance Comparison: AXI vs AHB
To quantify the AXI vs AHB performance difference, consider a system with one master and one slave. The table below compares their efficiency under different conditions.
Scenario | AXI Performance | AHB Performance |
---|---|---|
No Clock Delays, Single Transaction | Similar to AHB (single transaction) | Baseline performance |
Clock Delays, Outstanding Transactions | High throughput with multiple OTs | Lower throughput, sequential processing |
Dual-Port Slave, Read/Write | Up to 2x throughput (full duplex) | Limited to single transaction |
AXI’s ability to handle multiple outstanding transactions and duplex transfers makes it superior in most modern scenarios. However, AHB remains competitive in simple, low-latency systems.
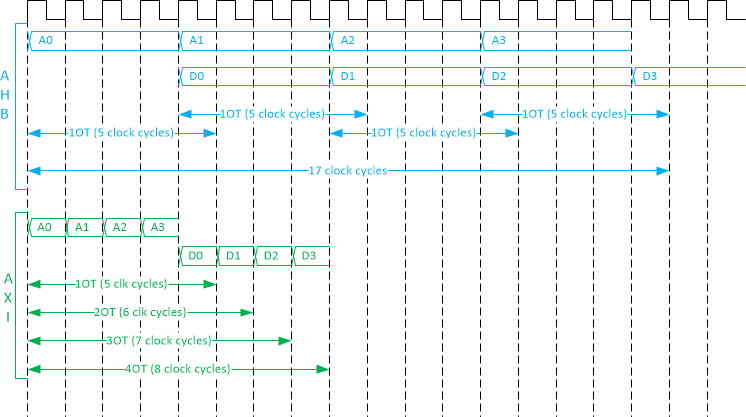
Additional Considerations
When deciding between AXI and AHB, consider these factors:
- Design Complexity: AXI’s multiple channels increase design and verification effort. AHB’s simpler structure reduces development time.
- Power Efficiency: AHB consumes less power, making it ideal for battery-powered devices.
- Scalability: AXI scales better for large SoCs with multiple masters and slaves.
Conclusion
The AXI vs AHB debate boils down to your project’s needs. AXI excels in high-performance, complex SoCs, offering superior throughput and flexibility. Its support for outstanding transactions, pipeline registers, and QoS makes it the go-to choice for modern applications. AHB, however, remains relevant for simpler, cost-sensitive, or legacy systems where low power and ease of implementation matter.
By understanding their differences and evaluating your requirements, you can confidently choose the right protocol. For hands-on learning, experiment with the provided Verilog code to see how AXI and AHB behave in your designs.